- Website
- Sabin Hertanu
How to connect Drizzle with PlanetScale in your Next.js app
PlanetScale is a MySQL-compatible serverless database, while Drizzle ORM is a TypeScript ORM that helps to interface with databases. The two have quickly become popular to use with full-stack applications built with Next.js. In this article, I'll show you how to set up and connect both in your Next.js app.
1. Install the required packages
First, we need to install the required packages. We'll need drizzle-orm
and @planetscale/database
to connect with PlanetScale. We'll also need drizzle-kit
to generate the database schema and dotenv
to load the environment variables. Assuming you've already created your Next.js app, run the following commands:
npm i drizzle-orm @planetscale/database
npm i -D drizzle-kit
npm i dotenv
2. Configure TypeScript
Drizzle does not yet support es5
and will throw the following error if you try to run it with the es5
target:
Transforming const to the configured target environment ("es5") is not supported yet.
In your tsconfig.json
, change the target
from es5
to es6
:
// tsconfig.json
{
"compilerOptions": {
"target": "es6"
}
}
3. Connect Drizzle with PlanetScale
Go to your database at https://app.planetscale.com/ and click the Connect button in the top-right corner.
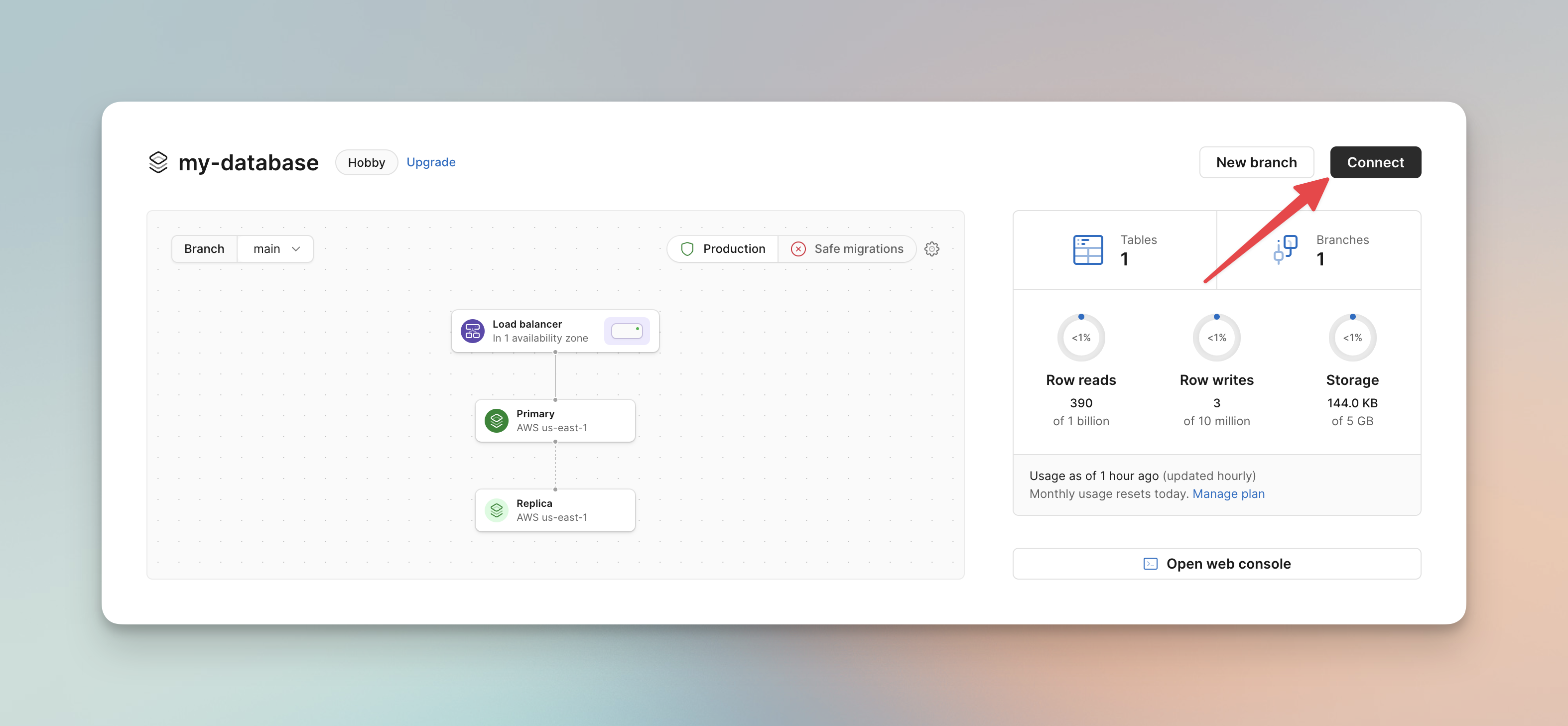
Pick the Drizzle option to connect with.
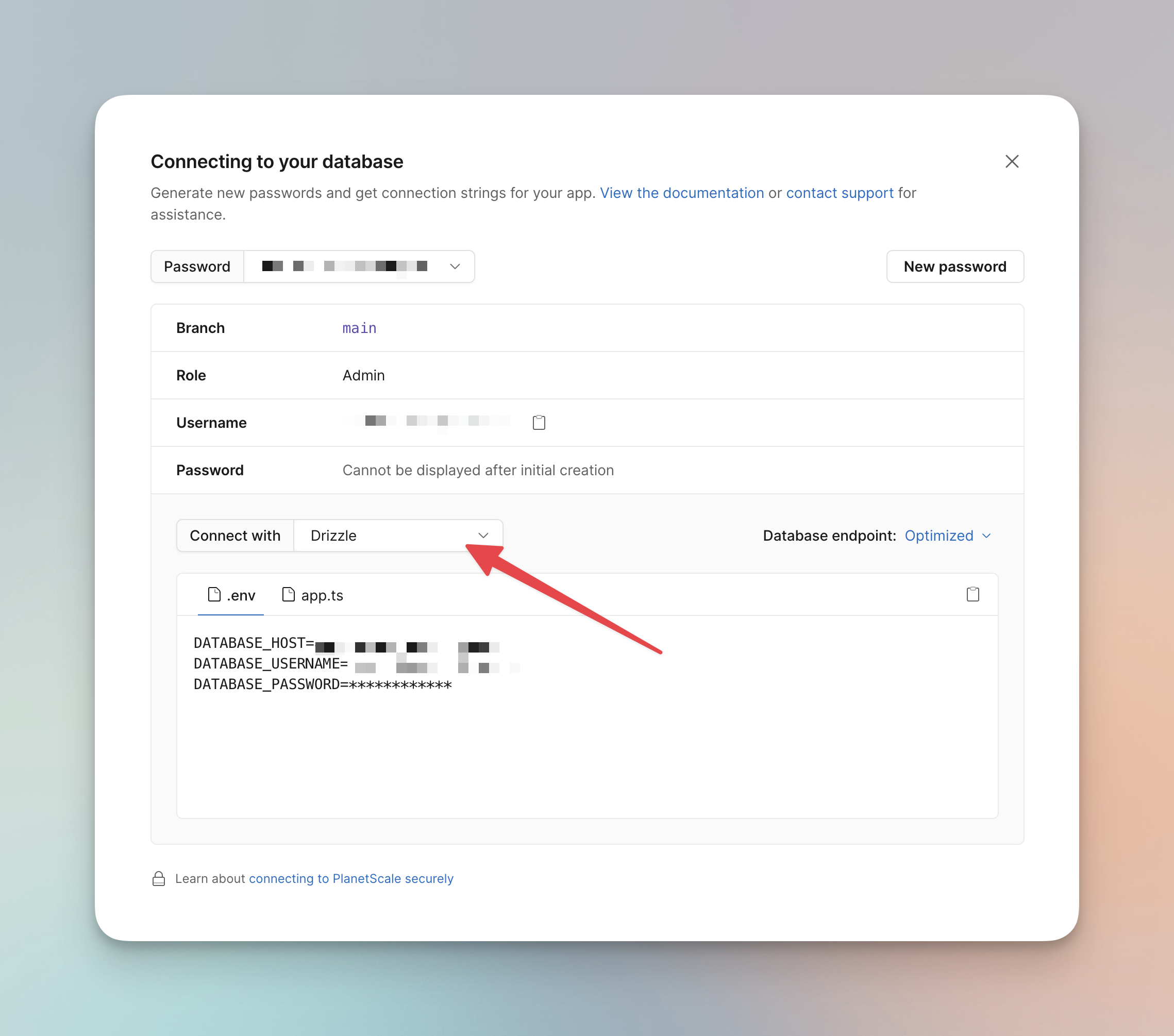
Copy the content of the .env
file and add it to your project's .env
file.
DATABASE_HOST=your-database-host
DATABASE_USERNAME=your-database-username
DATABASE_PASSWORD=************
Next click on the app.ts
tab and copy the code.
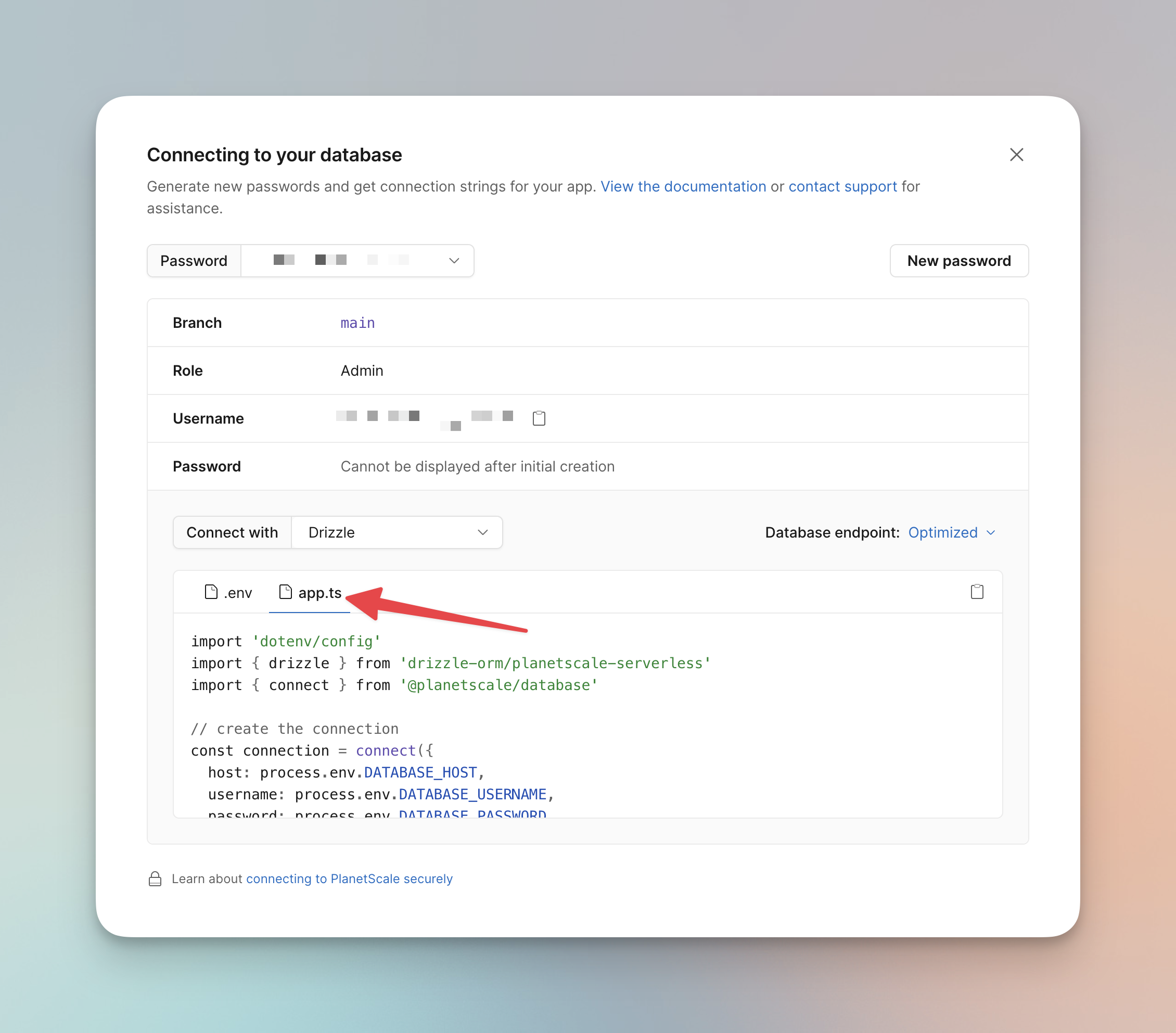
Go ahead and create a db/index.ts
file in the root of your project and add the code you just copied. You should end up with the following:
// db/index.ts
import 'dotenv/config'
import { drizzle } from 'drizzle-orm/planetscale-serverless'
import { connect } from '@planetscale/database'
// create the connection
const connection = connect({
host: process.env.DATABASE_HOST,
username: process.env.DATABASE_USERNAME,
password: process.env.DATABASE_PASSWORD,
})
export const db = drizzle(connection)
4. Configure Drizzle Kit
Next, we need to configure Drizzle Kit. Go back to your PlanetScale database connection settings, and this time select to connect with Prisma. We need to choose this to get the database URL. We need the database URL to make a slight modification to it.
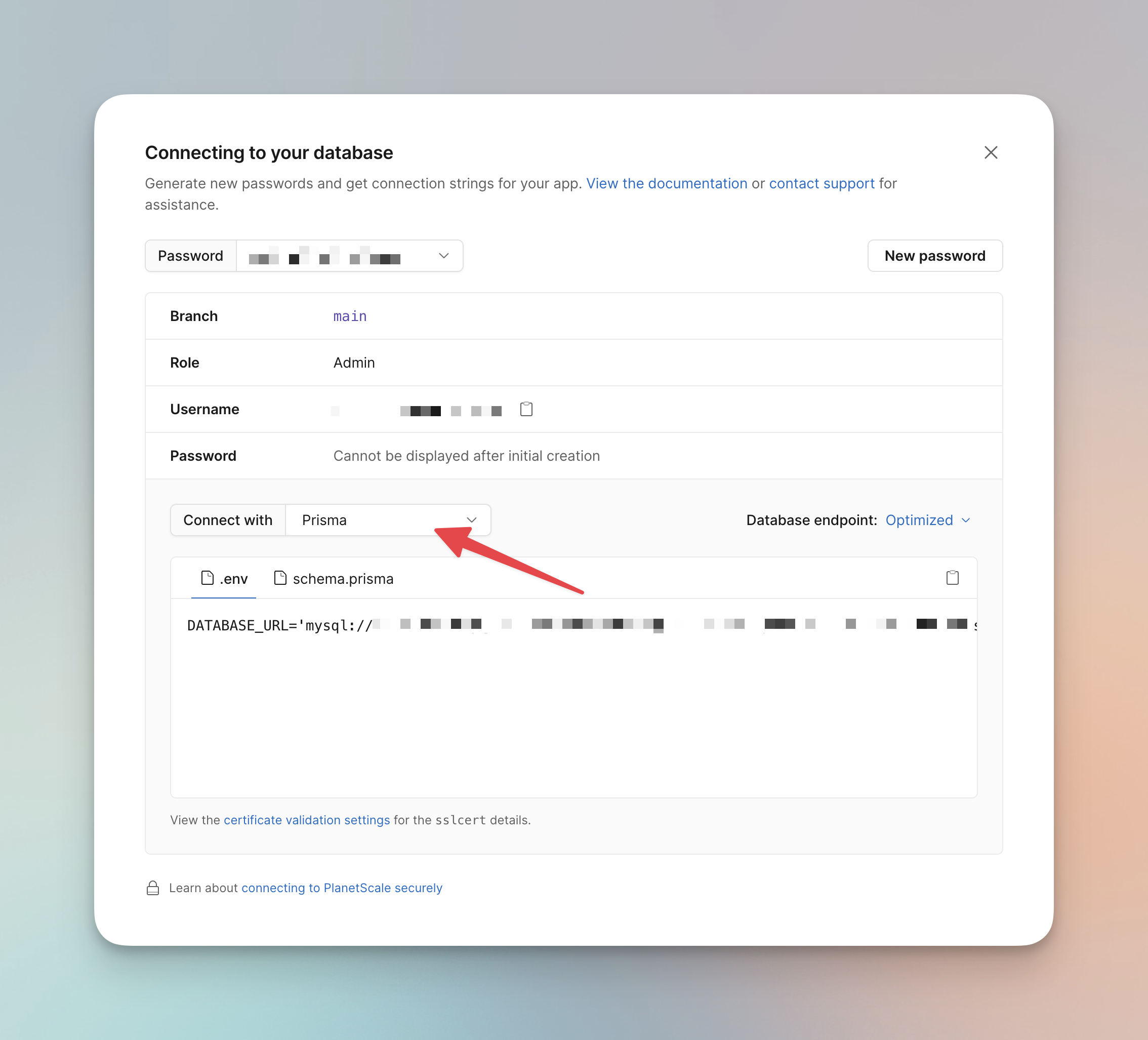
Copy the URL and change the last part ?sslaccept=strict
to ?ssl={"rejectUnauthorized":true}
. This change will allow you to connect to your PlanetScale database from your local machine. Copy this modified URL and add it to your .env
file. You should end up with the following:
DATABASE_URL='mysql://your-database-username:************@your-database-host/your-database-name?ssl={"rejectUnauthorized":true}'
Next, create a drizzle.config.ts
file in the root of your project and add the following code:
// drizzle.config.ts
import 'dotenv/config'
import type { Config } from 'drizzle-kit'
export default {
schema: './db/schema/*',
out: './drizzle',
driver: 'mysql2',
dbCredentials: {
uri: process.env.DATABASE_URL as string,
},
} satisfies Config
At this point, you can run local commands to connect to PlanetScale. Once you set up the database schema, you can run drizzle-kit push:mysql
to push it to PlanetScale.